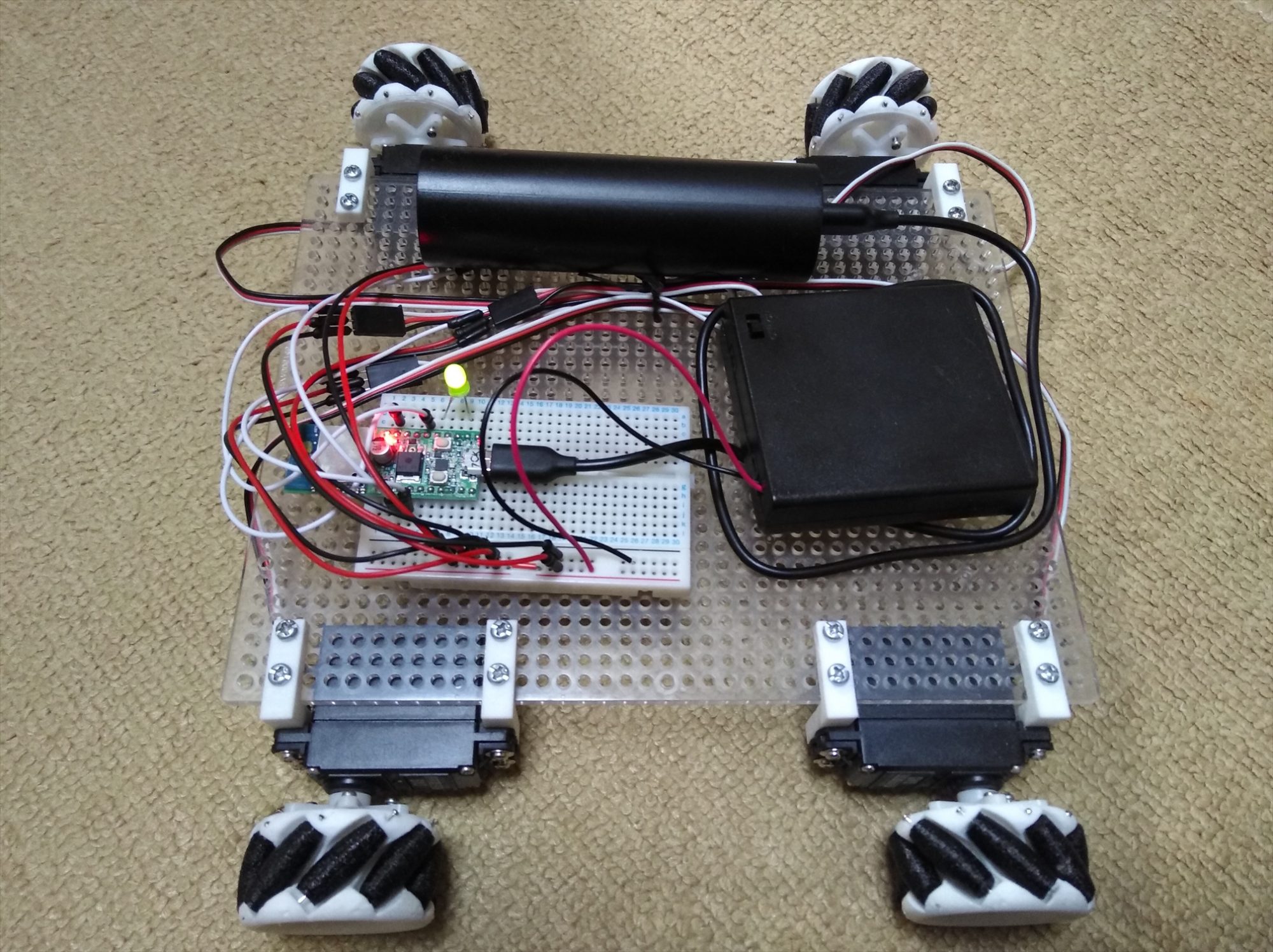
22,978 total views, 6 views today
先日、Arduino Uno互換機と赤外線リモコンを使うことによって、メカナムホイールのラジコンカーを作ることに成功した。
しかしながら、2メートルも離れてしまうと操作不能になってしまい、ラジコンとしては残念な感じである。
そこで、今度はWi-Fi越しにスマホなんかで操作できるようにすることにした。
見た目的には、Arduino互換機を使った赤外線ラジコン版とあまり変わらない。
オムニホイールのWi-Fiラジコンカーを3Dプリンターで自作した(ESP32)
Arduino Uno互換機を使ってメカナムホイールのラジコンカーを作った
動作
スマホで操作している様子
ESP-WROOM-02
ESP-WROOM-02は、Arduino IDEの利用が可能な、安価で小型のWi-Fiモジュールである。
Arduino IDEの利用が可能であることから、Arduino Unoとほぼ同じような感覚で、プログラムを組むことができる。
メカナムホイールのWi-Fiラジコン化にあたっては、この安価で導入が容易なESP-WROOM-02を使うことにした。
使用パーツ
使用するパーツについては、Arduino Uno互換機がESP-WROOM-02に置き換わったのと、LEDが1つ追加された以外は、基本的に前回の記事と同様である。
スポンサーリンク
配線
プログラム
#include <ESP8266WiFi.h> #include <WiFiClient.h> #include <ESP8266WebServer.h> #include <ESP8266mDNS.h> #include <Servo.h> const char* ssid = "nova"; const char* password = "novanova"; const int PIN_LED = 4; Servo F_R; Servo F_L; Servo B_R; Servo B_L; String html = ""; ESP8266WebServer server(80); void setup(void){ pinMode(PIN_LED, OUTPUT); digitalWrite(PIN_LED, LOW); F_R.attach(14); F_L.attach(12); B_R.attach(16); B_L.attach(5); html = "<!DOCTYPE html>\ <html>\ <head>\ <meta charset=\"UTF-8\">\ <meta name=\"viewport\" content=\"width=device-width,initial-scale=1,minimum-scale=1\">\ <style>\ table td{\ padding:15px;\ }\ </style>\ </head>\ <body>\ <br>\ <br>\ <br>\ <table>\ <tr><td><input type=\"button\" value=\"↖\" style=\"font-size:32px;\" onclick=\"location.href='/upper_left';\"></td><td><input type=\"button\" value=\"↑\" style=\"font-size:32px;\" onclick=\"location.href='/forward';\"></td><td><input type=\"button\" value=\"↗\" style=\"font-size:32px;\" onclick=\"location.href='/upper_right';\"></td></tr>\ <tr><td><input type=\"button\" value=\"←\" style=\"font-size:32px;\" onclick=\"location.href='/left';\"></td><td><input type=\"button\" value=\"■\" style=\"font-size:32px;\" onclick=\"location.href='/stop';\"></td><td><input type=\"button\" value=\"→\" style=\"font-size:32px;\" onclick=\"location.href='/right';\"></td></tr>\ <tr><td><input type=\"button\" value=\"↙\" style=\"font-size:32px;\" onclick=\"location.href='/down_left';\"></td><td><input type=\"button\" value=\"↓\" style=\"font-size:32px;\" onclick=\"location.href='/back';\"></td><td><input type=\"button\" value=\"↘\" style=\"font-size:32px;\" onclick=\"location.href='/down_right';\"></td></tr>\ </table>\ <br>\ <table>\ <tr><td><input type=\"button\" value=\"↺\" style=\"font-size:32px;\" onclick=\"location.href='/turn_left';\"></td><td><input type=\"button\" value=\"↻\" style=\"font-size:32px;\" onclick=\"location.href='/turn_right';\"></td></tr>\ </table>\ </body>\ </html>"; Serial.begin(115200); // WIFI_AP, WIFI_STA, WIFI_AP_STA or WIFI_OFF WiFi.mode(WIFI_STA); WiFi.begin(ssid, password); Serial.println(""); // Wifi接続ができるまで待機 while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.print("IPアドレス: "); Serial.println(WiFi.localIP()); // ローカルネットワーク内のみ有効のmDNS(マルチキャストDNS)を開始 // bool MDNSResponder::begin(const char* hostname){} if (MDNS.begin("petitmonte")) { Serial.println("mDNSレスポンダーの開始"); } // トップページ server.on("/", [](){ // HTTPステータスコード(200) リクエストの成功 server.send(200, "text/html", html); }); // 左斜め前方移動 server.on("/upper_left", [](){ UL(); server.send(200, "text/html", html); }); // 前進 server.on("/forward", [](){ FD(); server.send(200, "text/html", html); }); // 右斜め前方移動 server.on("/upper_right", [](){ UR(); server.send(200, "text/html", html); }); // 左移動 server.on("/left", [](){ SL(); server.send(200, "text/html", html); }); // 停止 server.on("/stop", [](){ ST(); server.send(200, "text/html", html); }); // 右移動 server.on("/right", [](){ SR(); server.send(200, "text/html", html); }); // 左斜め後方移動 server.on("/down_left", [](){ LL(); server.send(200, "text/html", html); }); // 後退 server.on("/back", [](){ BK(); server.send(200, "text/html", html); }); // 右斜め後方移動 server.on("/down_right", [](){ LR(); server.send(200, "text/html", html); }); // 左旋回 server.on("/turn_left", [](){ TL(); server.send(200, "text/html", html); }); // 右旋回 server.on("/turn_right", [](){ TR(); server.send(200, "text/html", html); }); // 存在しないURLを指定した場合の動作を指定する server.onNotFound([](){ // HTTPステータスコード(404) 未検出(存在しないファイルにアクセス) server.send(404, "text/plain", "404"); }); server.begin(); Serial.println("Webサーバーの開始"); digitalWrite(PIN_LED, HIGH); //Wifi接続したらLED点灯 } void loop(void){ // Webサーバの接続要求待ち server.handleClient(); } void UL() { Serial.println("upper left"); F_R.writeMicroseconds(0); F_L.writeMicroseconds(1500); B_R.writeMicroseconds(1500); B_L.writeMicroseconds(3000); } void FD() { Serial.println("forward"); F_R.writeMicroseconds(0); F_L.writeMicroseconds(3000); B_R.writeMicroseconds(0); B_L.writeMicroseconds(3000); } void UR() { Serial.println("upper right"); F_R.writeMicroseconds(1500); F_L.writeMicroseconds(3000); B_R.writeMicroseconds(0); B_L.writeMicroseconds(1500); } void SL() { Serial.println("sideways left"); F_R.writeMicroseconds(0); F_L.writeMicroseconds(0); B_R.writeMicroseconds(3000); B_L.writeMicroseconds(3000); } void ST() { Serial.println("stop"); F_R.writeMicroseconds(1500); F_L.writeMicroseconds(1500); B_R.writeMicroseconds(1500); B_L.writeMicroseconds(1500); } void SR() { Serial.println("sideways right"); F_R.writeMicroseconds(3000); F_L.writeMicroseconds(3000); B_R.writeMicroseconds(0); B_L.writeMicroseconds(0); } void LL() { Serial.println("lower left"); F_R.writeMicroseconds(1500); F_L.writeMicroseconds(0); B_R.writeMicroseconds(3000); B_L.writeMicroseconds(1500); } void BK() { Serial.println("backward"); F_R.writeMicroseconds(3000); F_L.writeMicroseconds(0); B_R.writeMicroseconds(3000); B_L.writeMicroseconds(0); } void LR() { Serial.println("lower right"); F_R.writeMicroseconds(3000); F_L.writeMicroseconds(1500); B_R.writeMicroseconds(1500); B_L.writeMicroseconds(0); } void TL() { Serial.println("turn left"); F_R.writeMicroseconds(0); F_L.writeMicroseconds(0); B_R.writeMicroseconds(0); B_L.writeMicroseconds(0); } void TR() { Serial.println("turn right"); F_R.writeMicroseconds(3000); F_L.writeMicroseconds(3000); B_R.writeMicroseconds(3000); B_L.writeMicroseconds(3000); }
まとめ
- 赤外線ラジコンのメカナムホイール車をWi-Fiラジコン化した
- Wi-Fiラジコン化には、ESP-WROOM-02を使用した
- 次は、オムニホイール車のWi-Fiラジコンを作る